In the ever-evolving landscape of web development, creating immersive and interactive 3D experiences has become increasingly popular. Three.js, a powerful JavaScript library, has emerged as a go-to tool for developers looking to bring three-dimensional graphics to the web. This comprehensive guide will walk you through the process of building interactive 3D websites using Three.js, from basic concepts to advanced techniques.
Introduction to Three.js
Three.js is an open-source JavaScript library that simplifies the process of creating and displaying animated 3D computer graphics in a web browser. It provides a high-level API for WebGL, making it accessible even to developers who may not have extensive experience with 3D graphics programming.
Setting Up Your Development Environment
Before diving into Three.js, you’ll need to set up your development environment. Start by including the Three.js library in your project. You can either download it directly or use a content delivery network (CDN):
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
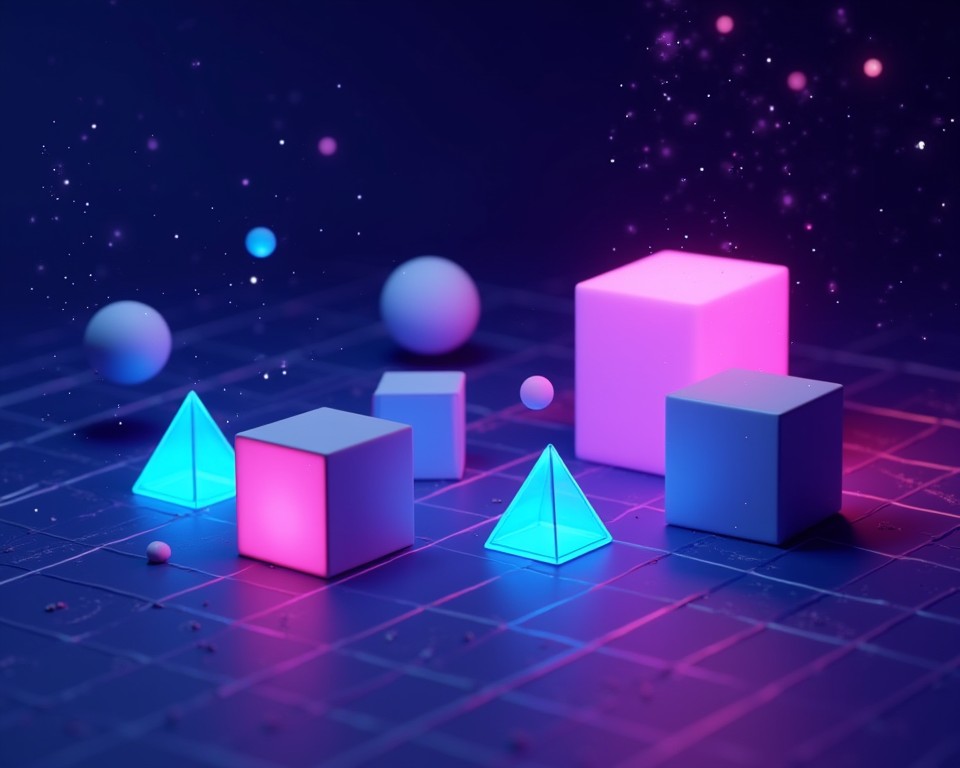
Creating Your First 3D Scene
To create a basic 3D scene with Three.js, you\’ll need three essential components:
- A scene to hold all your objects
- A camera to determine what’s visible
- A renderer to display your scene
Here\’s a simple example to get you started:
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
camera.position.z = 5;
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
This code creates a simple green cube that rotates continuously.
Adding Interactivity
To make your 3D website truly engaging, you\’ll want to add interactivity. Three.js provides several ways to handle user input:
- Mouse Events: Use the
THREE.Raycaster
to detect when the mouse intersects with 3D objects. - Keyboard Controls: Implement keyboard controls to move objects or the camera.
- OrbitControls: This built-in control allows users to orbit around a target point.
Here\’s an example of implementing OrbitControls:
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls.js';
const controls = new OrbitControls(camera, renderer.domElement);
controls.enableDamping = true;
controls.dampingFactor = 0.25;
controls.enableZoom = true;
function animate() {
requestAnimationFrame(animate);
controls.update();
renderer.render(scene, camera);
}
Optimizing Performance
As your 3D scenes become more complex, optimizing performance becomes crucial. Here are some tips:
- Use
THREE.BufferGeometry
instead ofTHREE.Geometry
for better performance. - Implement level of detail (LOD) for complex objects.
- Use texture atlases to reduce draw calls.
- Utilize object pooling for frequently created and destroyed objects.
Advanced Techniques
Once you’re comfortable with the basics, you can explore advanced techniques:
- Shaders: Create custom materials using GLSL shaders for unique visual effects.
- Particle Systems: Generate impressive effects like fire, smoke, or stars.
- Physics: Integrate a physics engine like Cannon.js for realistic object interactions.
- VR and AR: Explore virtual and augmented reality possibilities with Three.js.
Conclusion
Building interactive 3D websites with Three.js opens up a world of creative possibilities. From product visualizations to immersive games, the applications are limitless. As you continue to explore and experiment with Three.js, you\’ll discover new ways to push the boundaries of what\’s possible on the web.
Remember, the key to mastering Three.js is practice and experimentation. Start with simple projects and gradually increase complexity as you become more comfortable with the library. Happy coding, and may your 3D creations inspire and amaze!